Lecture 1
Reading: Textbook Chapter 1
Contents:
What is a program and why develop program?
What is a programming language?
Brief introduction of a system development life cycle
Steps in program development
Writing, editing, compiling and linking program
Program execution
What is a program?
- A program is a set of instructions for the computer to execute.
Why develop program?
- We develop program because we want to instruct the computer to do jobs
for us.
What is a programming language?
- fig. 1-8
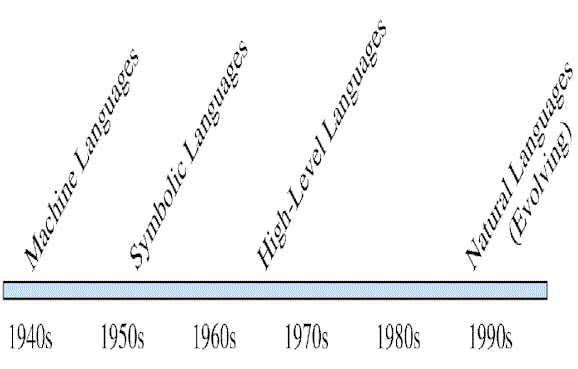
- We use a programming language to write a computer program.
- The only language understood directly by a computer is machine
language, which consists of 0s and 1s only.
- Every computer has its own set of machine language.
- Symbolic languages: very similar to machine languages except that
mnemonics are used to replace the numbers.
- High-level languages: programming languages that are relatively
easy to be understood by human.
- High-level languages are portable to many different computers.
- Today the most popular high-level language is C – the language to be
learnt in this subject.
- Symbolic languages and high-level languages must be translated into
machine languages before they can be executed by the computer.
- Research on using natural languages as programming languages is
being done.
Brief introduction of a system development life cycle
fig. 1-11
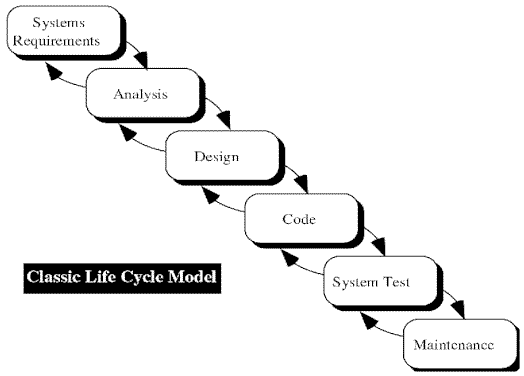
- Programming projects are built using a series of interrelated phases
referred to as the system development life cycle.
- Although the exact number and names of the phases differ depending on the
environment, there is general agreement as to the steps that must be followed.
- A life cycle model may have the following phases:
Systems
requirement: defines requirements that specify what the proposed system is to
accomplish.
Analysis: looks at different alternatives.
Design: determines how the system will be built; functions of
individual programs and the design of the files / databases is completed.
Code: writing programs – focus of this subject.
System test: all the programs are tested together to make sure
the system works as a whole.
Maintenance: keeps the system working once it has been put into
production.
- Phases may need to be reworked before entering the next phases.
Steps in program development
- Understand the problem.
- Develop the solution: tools include structure chart, pseudocode,
and flowchart (using either pseudocode or flowchart will be sufficient).
fig. 1-12
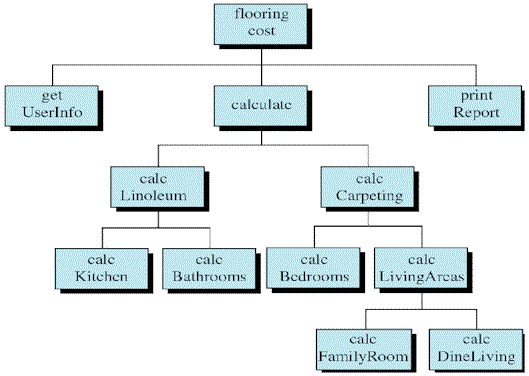
-
- Structure chart: shows functional flow through the program; i.e.
shows interaction between all the parts (modules) of the program.
- Pseudocode: part English, part program logic; describes the
algorithm in detail and can be converted to a program easily.
Pseudocode example:
to calculate the number of male and female students
by reading a file:
set maleCount to 0
set femaleCount to 0
read the first record from the student file
while not end of file
if the gender of the
student is male
add 1 to maleCount
else
add 1 to
femaleCount
read the next record from the student file
output the maleCount
output the femaleCount
|
Flowchart: similar to pseudocode except that diagrams are used to
indicate the flow.
fig. 1-13
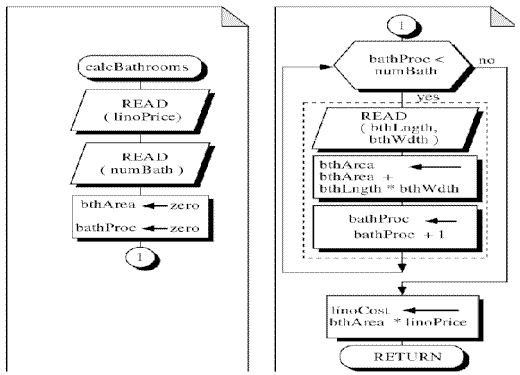
- Write the program: converting the pseudocode or flowchart into
programming language.
- Test the program: feed in sufficient test data to make sure that
the program works in every case.
Writing, editing, compiling and linking program
fig. 1-9
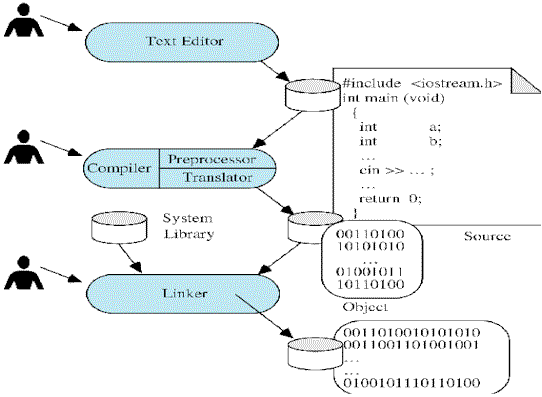
- The software that we use to write and edit our programs is the text
editor.
- A text editor lets you enter, change, and store character data.
- The saved program file is called the source file.
- A compiler is used to convert the source file into machine
language.
- The C compiler consists of the preprocessor and the translator.
- The preprocessor scans for preprocessor directives which tell it to look
for special code libraries, make substitutions in the program code, etc.
- The translator converts the output of the preprocessor into machine
language, the result being called object module.
- The linker combines the program functions and the library
functions into an executable program, which is ready to be run.
Program execution
fig. 1-10
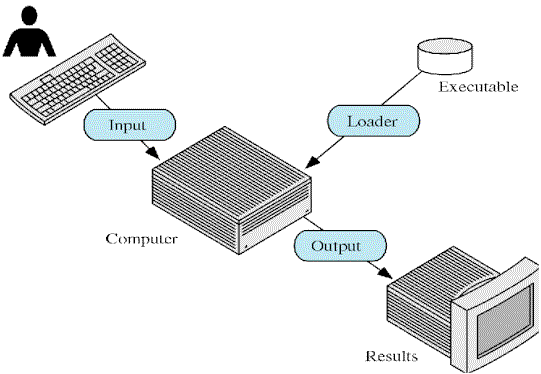
- To execute the program an operating system command is issued to load the
program into primary memory and then execute it.
- Typically, the program reads in data for processing, processes them, and
generates the output; the program is then removed from memory.
- End of Lecture 1-